Java Generate Key Pair Programmatically
If a code signer does not yet have a suitable private key for signing the code, the key must first be generated, along with a corresponding public key that can be used by the code receiver's runtime system to verify the signature.
In this quickstart, you've learned how to create an Azure Cosmos DB SQL API account, create a document database and container using the Data Explorer, and run a Java app to do the same thing programmatically. You can now import additional data into your Azure Cosmos DB account. You can use it to generate a certificate from your key pair and insert it into the certificate chain in order to make the setKeyEntry method work: Don't specify SHA1PRNG, instead let the system choose the random number provider for you. The Java Keytool is a command line tool which can generate public key / private key pairs and store them in a Java KeyStore.The Keytool executable is distributed with the Java SDK (or JRE), so if you have an SDK installed you will also have the Keytool executable.
Since this lesson assumes that you don't yet have such keys, you are going to create a keystore named examplestore
and create an entry with a newly generated public/private key pair (with the public key in a certificate).
The genkey command of the keytool program enables you to generate a key pair. To Generate a Key Pair and a Self-Signed Certificate Navigate to the JAVAHOME/bin directory, where JAVAHOME is the installation directory of the Java SDK. I'm thinking to generate the keypair at HSM. Extract out the public key from HSM and the private key remain store at HSM. Then, i will use the public key to generate the CSR and then let the HSM sign the CSR using the private key and then make the whole thing to conform to PKCS#10 standard. I see you've already gone over to the BouncyCastle side of the house but just in case anyone else was wondering; you can add the cert chain to the entry when putting the key into the KeyStore.
Type the following command in your command window to create a keystore named examplestore
and to generate keys:
You will be prompted to enter passwords for the key and keystore.
Subparts of the keytool Command
Let's look at what each of the keytool
subparts mean.
- The command for generating keys is -genkey.
- The -alias signFiles subpart indicates the alias to be used in the future to refer to the keystore entry containing the keys that will be generated.
- The -keystore examplestore subpart indicates the name (and optionally path) of the keystore you are creating or already using.
- The storepass value that you are promted for specifies the keystore password.
- The keypass value that you are prompted for specifies a password for the private key about to be generated. You will always need this password in order to access the keystore entry containing that key. The entry doesn't have to have its own password. When you are prompted for the key password, you are given the option of letting it be the same as the keystore password.
Note: For security reasons you should not set your key or keystore passwords on the command line, because they can be intercepted more easily that way.
Distinguished-Name Information
If you use the preceding keystore
command, you will be prompted for your distinguished-name information. Following are the prompts; the bold indicates what you should type.
Command Results
The keytool
command creates the keystore named examplestore
(if it doesn't already exist) in the same directory in which the command is executed. The command generates a public/private key pair for the entity whose distinguished name has a common name of Susan Jones and the organizational unit of Purchasing.
The command creates a self-signed certificate that includes the public key and the distinguished-name information. (The distinguished name you supply will be used as the 'subject' field in the certificate.) This certificate will be valid for 90 days, the default validity period if you don't specify a -validity option. The certificate is associated with the private key in a keystore entry referred to by the alias signFiles
.
Self-signed certificates are useful for developing and testing an application. However, users are warned that the application is signed with an untrusted certificate and asked if they want to run the application. To provide users with more confidence to run your application, use a certificate issued by a recognized certificate authority.
Note: The command could be shorter if option defaults are accepted or you wish to be prompted for various values. Whenever you execute a keytool
command, defaults are used for unspecified options that have default values, and you are prompted for any required values. For the genkey
command, options with default values include alias (whose default is mykey
), validity (90 days), and keystore (the file named .keystore
in your home directory). Required values include dname, storepass, and keypass.
In this quickstart, you create and manage an Azure Cosmos DB SQL API account from the Azure portal, and by using a Java app cloned from GitHub. First, you create an Azure Cosmos DB SQL API account using the Azure portal, then create a Java app using the SQL Java SDK, and then add resources to your Cosmos DB account by using the Java application. Azure Cosmos DB is a multi-model database service that lets you quickly create and query document, table, key-value, and graph databases with global distribution and horizontal scale capabilities.
Prerequisites
- An Azure account with an active subscription. Create one for free. Or try Azure Cosmos DB for free without an Azure subscription. You can also use the Azure Cosmos DB Emulator with a URI of
https://localhost:8081
and the keyC2y6yDjf5/R+ob0N8A7Cgv30VRDJIWEHLM+4QDU5DE2nQ9nDuVTqobD4b8mGGyPMbIZnqyMsEcaGQy67XIw/Jw
. - Java Development Kit (JDK) 8. Point your
JAVA_HOME
environment variable to the folder where the JDK is installed. - A Maven binary archive. On Ubuntu, run
apt-get install maven
to install Maven. - Git. On Ubuntu, run
sudo apt-get install git
to install Git.
Introductory notes
The structure of a Cosmos DB account. Irrespective of API or programming language, a Cosmos DB account contains zero or more databases, a database (DB) contains zero or more containers, and a container contains zero or more items, as shown in the diagram below:
You may read more about databases, containers and items here. A few important properties are defined at the level of the container, among them provisioned throughput and partition key.
The provisioned throughput is measured in Request Units (RUs) which have a monetary price and are a substantial determining factor in the operating cost of the account. Provisioned throughput can be selected at per-container granularity or per-database granularity, however container-level throughput specification is typically preferred. You may read more about throughput provisioning here.
As items are inserted into a Cosmos DB container, the database grows horizontally by adding more storage and compute to handle requests. Storage and compute capacity are added in discrete units known as partitions, and you must choose one field in your documents to be the partition key which maps each document to a partition. The way partitions are managed is that each partition is assigned a roughly equal slice out of the range of partition key values; therefore you are advised to choose a partition key which is relatively random or evenly-distributed. Otherwise, some partitions will see substantially more requests (hot partition) while other partitions see substantially fewer requests (cold partition), and this is to be avoided. You may learn more about partitioning here.
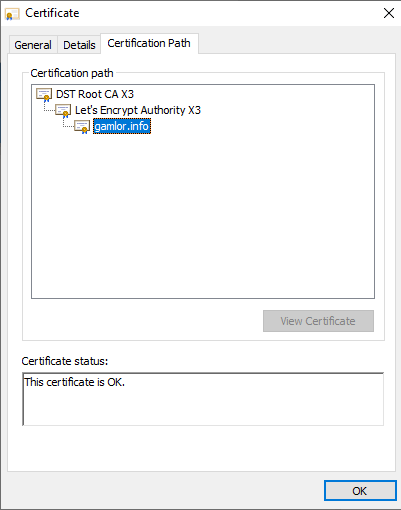
Java Generate Key Pair
Create a database account
Before you can create a document database, you need to create a SQL API account with Azure Cosmos DB.
Go to the Azure portal to create an Azure Cosmos DB account. At your homepage choose Create a resource from the Azure services panel.
Search for and select Azure Cosmos DB.
Select Create.
On the Create Azure Cosmos DB Account page, enter the basic settings for the new Azure Cosmos account.
Setting Value Description Subscription Subscription name Select the Azure subscription that you want to use for this Azure Cosmos account. Resource Group Resource group name Select a resource group, or select Create new, then enter a unique name for the new resource group. Account Name A unique name Enter a name to identify your Azure Cosmos account. Because documents.azure.com is appended to the name that you provide to create your URI, use a unique name.
The name can only contain lowercase letters, numbers, and the hyphen (-) character. It must be between 3-31 characters in length.API The type of account to create Select Core (SQL) to create a document database and query by using SQL syntax.
The API determines the type of account to create. Azure Cosmos DB provides five APIs: Core (SQL) and MongoDB for document data, Gremlin for graph data, Azure Table, and Cassandra. Currently, you must create a separate account for each API.
Learn more about the SQL API.Apply Free Tier Discount Apply or Do not apply With Azure Cosmos DB free tier, you will get the first 400 RU/s and 5 GB of storage for free in an account. Learn more about free tier. Location The region closest to your users Select a geographic location to host your Azure Cosmos DB account. Use the location that is closest to your users to give them the fastest access to the data. Account Type Production or Non-Production Select Production if the account will be used for a production workload. Select Non-Production if the account will be used for non-production, e.g. development, testing, QA, or staging. This is an Azure resource tag setting that tunes the Portal experience but does not affect the underlying Azure Cosmos DB account. You can change this value anytime. Note
You can have up to one free tier Azure Cosmos DB account per Azure subscription and must opt-in when creating the account. If you do not see the option to apply the free tier discount, this means another account in the subscription has already been enabled with free tier.
Select Review + create. You can skip the Network and Tags sections. Gpg generate public key.
Review the account settings, and then select Create. It takes a few minutes to create the account. Wait for the portal page to display Your deployment is complete.
Select Go to resource to go to the Azure Cosmos DB account page.
Add a container
You can now use the Data Explorer tool in the Azure portal to create a database and container.
Select Data Explorer > New Container.
The Add Container area is displayed on the far right, you may need to scroll right to see it.
In the Add container page, enter the settings for the new container.
Setting Suggested value Description Database ID Tasks Enter Tasks as the name for the new database. Database names must contain from 1 through 255 characters, and they cannot contain /, , #, ?
, or a trailing space. Check the Provision database throughput option, it allows you to share the throughput provisioned to the database across all the containers within the database. This option also helps with cost savings.Throughput 400 Leave the throughput at 400 request units per second (RU/s). If you want to reduce latency, you can scale up the throughput later. Container ID Items Enter Items as the name for your new container. Container IDs have the same character requirements as database names. Partition key /category The sample described in this article uses /category as the partition key. In addition to the preceding settings, you can optionally add Unique keys for the container. Let's leave the field empty in this example. Unique keys provide developers with the ability to add a layer of data integrity to the database. By creating a unique key policy while creating a container, you ensure the uniqueness of one or more values per partition key. To learn more, refer to the Unique keys in Azure Cosmos DB article.
Select OK. The Data Explorer displays the new database and container.
Add sample data
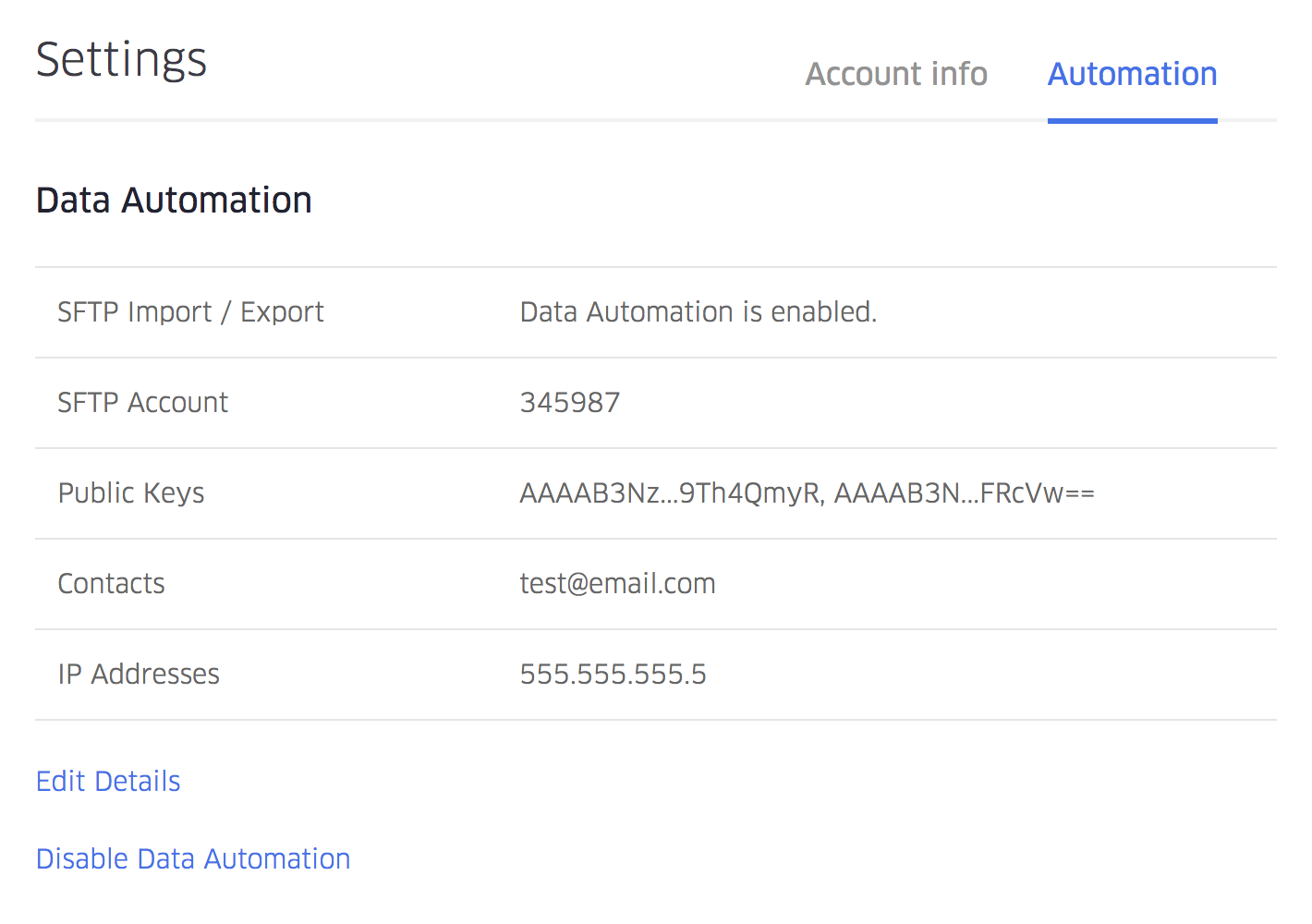
You can now add data to your new container using Data Explorer.
From the Data Explorer, expand the Tasks database, expand the Items container. Select Items, and then select New Item.
Now add a document to the container with the following structure.
Once you've added the json to the Documents tab, select Save.
Create and save one more document where you insert a unique value for the
id
property, and change the other properties as you see fit. Your new documents can have any structure you want as Azure Cosmos DB doesn't impose any schema on your data.
Query your data
You can use queries in Data Explorer to retrieve and filter your data.
At the top of the Items tab in Data Explorer, review the default query
SELECT * FROM c
. This query retrieves and displays all documents from the container ordered by ID.To change the query, select Edit Filter, replace the default query with
ORDER BY c._ts DESC
, and then select Apply Filter.The modified query displays the documents in descending order based on their time stamp, so now your second document is listed first.
If you're familiar with SQL syntax, you can enter any supported SQL queries in the query predicate box. You can also use Data Explorer to create stored procedures, UDFs, and triggers for server-side business logic.
Data Explorer provides easy Azure portal access to all of the built-in programmatic data access features available in the APIs. You also use the portal to scale throughput, get keys and connection strings, and review metrics and SLAs for your Azure Cosmos DB account.
Clone the sample application
Now let's switch to working with code. Let's clone a SQL API app from GitHub, set the connection string, and run it. You'll see how easy it is to work with data programmatically.
Run the following command to clone the sample repository. This command creates a copy of the sample app on your computer.
Review the code
This step is optional. If you're interested in learning how the database resources are created in the code, you can review the following snippets. Otherwise, you can skip ahead to Run the app.
Managing database resources using the synchronous (sync) API
CosmosClient
initialization. TheCosmosClient
provides client-side logical representation for the Azure Cosmos database service. This client is used to configure and execute requests against the service.CosmosDatabase
creation.CosmosContainer
creation.Item creation by using the
createItem
method.Point reads are performed using
readItem
method.SQL queries over JSON are performed using the
queryItems
method.
Managing database resources using the asynchronous (async) API
Async API calls return immediately, without waiting for a response from the server. In light of this, the following code snippets show proper design patterns for accomplishing all of the preceding management tasks using async API.
CosmosAsyncClient
initialization. TheCosmosAsyncClient
provides client-side logical representation for the Azure Cosmos database service. This client is used to configure and execute asynchronous requests against the service.CosmosAsyncDatabase
creation.CosmosAsyncContainer
creation.As with the sync API, item creation is accomplished using the
createItem
method. This example shows how to efficiently issue numerous asynccreateItem
requests by subscribing to a Reactive Stream which issues the requests and prints notifications. Since this simple example runs to completion and terminates,CountDownLatch
instances are used to ensure the program does not terminate during item creation. The proper asynchronous programming practice is not to block on async calls - in realistic use-cases requests are generated from a main() loop that executes indefinitely, eliminating the need to latch on async calls.As with the sync API, point reads are performed using
readItem
method.As with the sync API, SQL queries over JSON are performed using the
queryItems
method.
Run the app
Now go back to the Azure portal to get your connection string information and launch the app with your endpoint information. This enables your app to communicate with your hosted database.
In the git terminal window,
cd
to the sample code folder.In the git terminal window, use the following command to install the required Java packages.
In the git terminal window, use the following command to start the Java application (replace SYNCASYNCMODE with
sync
orasync
depending on which sample code you would like to run, replace YOUR_COSMOS_DB_HOSTNAME with the quoted URI value from the portal, and replace YOUR_COSMOS_DB_MASTER_KEY with the quoted primary key from portal)The terminal window displays a notification that the FamilyDB database was created.
The app creates database with name
AzureSampleFamilyDB
The app creates container with name
FamilyContainer
The app will perform point reads using object IDs and partition key value (which is lastName in our sample).
The app will query items to retrieve all families with last name in ('Andersen', 'Wakefield', 'Johnson')
The app doesn't delete the created resources. Switch back to the portal to clean up the resources. from your account so that you don't incur charges.
Review SLAs in the Azure portal
The Azure portal monitors your Cosmos DB account throughput, storage, availability, latency, and consistency. Charts for metrics associated with an Azure Cosmos DB Service Level Agreement (SLA) show the SLA value compared to actual performance. This suite of metrics makes monitoring your SLAs transparent.
To review metrics and SLAs:
Java Generate Key Pair Programmatically List
Select Metrics in your Cosmos DB account's navigation menu.
Select a tab such as Latency, and select a timeframe on the right. Compare the Actual and SLA lines on the charts.
Review the metrics on the other tabs.
Clean up resources
When you're done with your app and Azure Cosmos DB account, you can delete the Azure resources you created so you don't incur more charges. To delete the resources:
In the Azure portal Search bar, search for and select Resource groups.
From the list, select the resource group you created for this quickstart.
On the resource group Overview page, select Delete resource group.
In the next window, enter the name of the resource group to delete, and then select Delete.
Next steps
Generate Key Code
In this quickstart, you've learned how to create an Azure Cosmos DB SQL API account, create a document database and container using the Data Explorer, and run a Java app to do the same thing programmatically. You can now import additional data into your Azure Cosmos DB account.